I’m not trying to beat a dead horse here—I understand your stance after reading through this post. However, I have a suggestion: could you add a calculation on the invoice page showing the exact amount a client would need to pay to cover the Stripe processing fee?
I don’t have many clients who pay by card, but for the few who do, they’re happy to cover the fee in exchange for the convenience of not writing a check. If the system displayed this calculation, I could simply add a line item for the fee, making it easier for both me and my clients.
I get that it may not be worth the extra coding effort, but to me, this feels like a good compromise. It keeps the default stance unchanged while still accommodating those clients who don’t mind paying extra for the convenience.
The calculation would be straightforward:
If a client wants to pay $100 via Stripe, the total charge should account for Stripe’s 2.9% + $0.30 processing fee. The formula to determine the correct amount is:
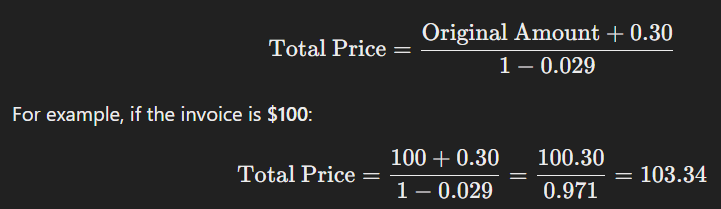
So, the client would need to pay $103.34 for you to receive exactly $100 after Stripe’s fees.
Would this be possible to implement? It wouldn’t automatically add the fee, but it would at least provide the correct calculation for those clients who prefer to pay by card.
ChatGPT code but surely it would be possible
<?php
// Function to calculate total amount including Stripe fee
function calculateStripeTotal($amount) {
$stripe_fee_percentage = 0.029; // 2.9%
$stripe_fixed_fee = 0.30; // $0.30 per transaction
// Formula to get the correct total amount
$total_with_fee = ($amount + $stripe_fixed_fee) / (1 - $stripe_fee_percentage);
return number_format($total_with_fee, 2, '.', '');
}
// Example: Fetch invoice amount dynamically
$invoice_total = 100.00; // Replace this with the actual invoice total
$stripe_total = calculateStripeTotal($invoice_total);
?>
<!-- Display the Stripe-adjusted amount on the invoice page -->
<div class="invoice-payment-info">
<p><strong>Original Invoice Total:</strong> $<?php echo number_format($invoice_total, 2); ?></p>
<p><strong>Amount to Cover Stripe Fee:</strong> $<?php echo $stripe_total; ?></p>
<p>(This ensures you receive exactly $<?php echo number_format($invoice_total, 2); ?> after Stripe fees.)</p>
</div>